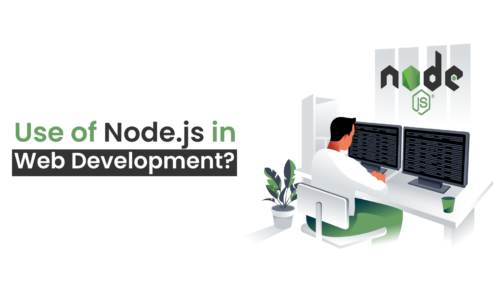
Module 1: JavaScript Fundamentals
- Introduction to JavaScript – History and Importance
- Setting up a JavaScript Development Environment
- Variables, Data Types, and Basic Operators
- Conditional Statements: If, Else, and Switch
- Loops: For, While, and Do-While
- Functions: Declaration, Expressions, and Arrow Functions
- Objects and Arrays: Basics
- Event Handling in JavaScript
- Error Handling and Debugging
- Closures and Scopes
Module 2: Asynchronous JavaScript
- Understanding Synchronous vs. Asynchronous Programming
- Callbacks: Handling Asynchronous Operations
- Promises: A Better Way to Handle Asynchrony
- Async/Await: Modern Asynchronous Code
- Working with AJAX and Fetch API
- Timers: SetTimeout and SetInterval
- Handling Multiple Promises with Promise.all()
- Error Handling in Asynchronous Code
- Introduction to WebSockets
- Asynchronous Iterators and Generators
Module 3: Working with the Document Object Model (DOM)
- Introduction to the DOM: What is it?
- Selecting and Manipulating Elements
- Creating and Removing Elements
- Traversing the DOM: Parents, Children, and Siblings
- Working with Attributes and Classes
- Handling DOM Events: Listeners and Event Objects
- Form Handling and Validation
- Using Data Attributes and Custom Properties
- Effects and Animations
- Best Practices for DOM Manipulation
Module 4: Introduction to Node.js
- What is Node.js? An Overview
- Setting up Node.js: Installation and Environment
- Understanding the Node.js Event Loop
- Modules and NPM: Node.js Ecosystem
- Working with File System in Node.js
- Streams and Buffers
- Handling HTTP Requests with Node.js
- Building a Basic HTTP Server
- Introduction to the Node Package Manager (NPM)
- Debugging Node.js Applications
Module 5: Building Web Applications with Express.js
- Introduction to Express.js
- Setting up an Express Application
- Routes and Middleware
- Working with Templates and Views
- Handling Forms and File Uploads
- Databases Integration with Express
- User Authentication and Sessions
- Error Handling and Debugging in Express
- Securing Express Applications
- Deploying Express Applications